Arrays in R Programming
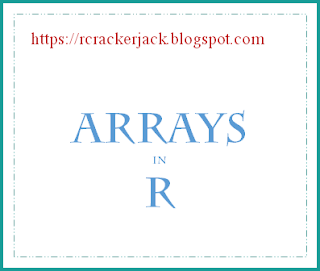
DATA TYPE: Graphical Representation of Data Types in R is shown as below: ARRAY: An array is created using the array() function. It is Multi-Dimensional. Syntax: array(data = NA, dim = length(data), dimnames = NULL) Data - Can be a Vector dim- c(x,y,k); x indicates the number of rows, y indicates the number of columns, k denotes the number of Matrices dimnames- NULL or List 1. Single Dimensional Array a1<-array(1:10) a1 [1] 1 2 3 4 5 6 7 8 9 10 class(a1) [1] "array" 2. Two Dimensional Array a2<-array(1:10,dim=c(5,2)) a2 [,1] [,2] [1,] 1 6 [2,] 2 7 [3,] 3...